➤ How to Code a Game
➤ Array Programs in Java
➤ Java Inline Thread Creation
➤ Java Custom Exception
➤ Hibernate vs JDBC
➤ Object Relational Mapping
➤ Check Oracle DB Size
➤ Check Oracle DB Version
➤ Generation of Computers
➤ XML Pros & Cons
➤ Git Analytics & Its Uses
➤ Top Skills for Cloud Professional
➤ How to Hire Best Candidates
➤ Scrum Master Roles & Work
➤ CyberSecurity in Python
➤ Protect from Cyber-Attack
➤ Solve App Development Challenges
➤ Top Chrome Extensions for Twitch Users
➤ Mistakes That Can Ruin Your Test Metric Program
Swagger with Spring Boot REST | POSTMAN Tool tests our rest controllers. This is a fully manual tool, we should enter all details: URL, MethodType, Param, JSON Input, Header Details, etc.
We should list all URLs in the application, if it is a small application then it is fine. But if the application is big (having 40 controllers with multiple methods like save, update, delete, get, etc) in this case checking all URLs, types, and Input is a bit complex and time-consuming process.
Swagger is an advanced test tool compared to POSTMAN. It provides an easy UI for testing all RestControllers in the project.
If we use Swagger, we do need not to enter the URL, and choose Method Type manually. We need to enter only input data.
Swagger Configuration in Spring Boot REST
Step-1:- Define one Spring Boot project with RestControllers
Step-2:- Add the springdoc-openapi-starter-webmvc-ui
dependencies
<dependency>
<groupId>org.springdoc</groupId>
<artifactId>springdoc-openapi-starter-webmvc-ui</artifactId>
<version>2.3.0</version>
</dependency>
See more:- Why not to use springfox-swagger2, and springfox-swagger-ui dependecies
We don’t need to add any configuration, no need to create the Docket, and no need to give the rest controller package path.
The Swagger UI page will then be available at:- http://localhost:8080/swagger-ui/index.html
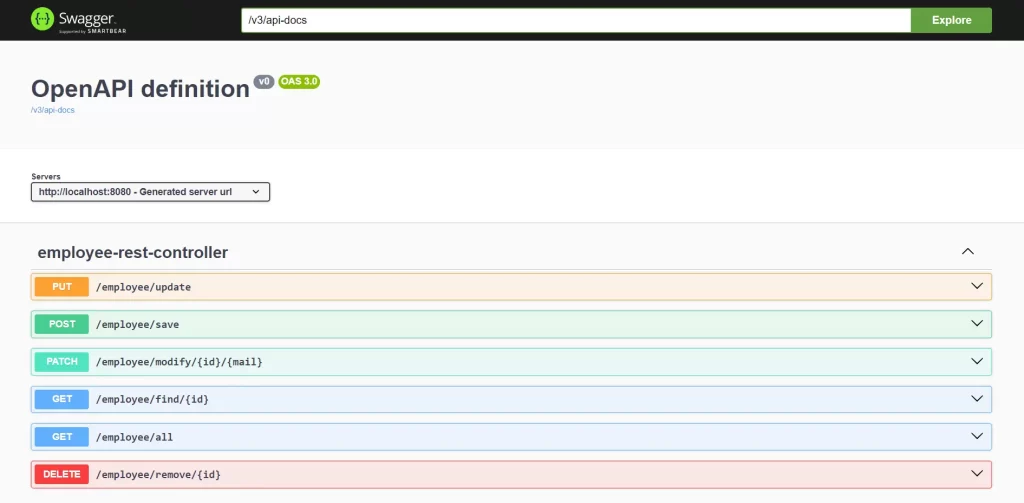
Swagger is not for adding any functionalities, it works only to make testing easy.
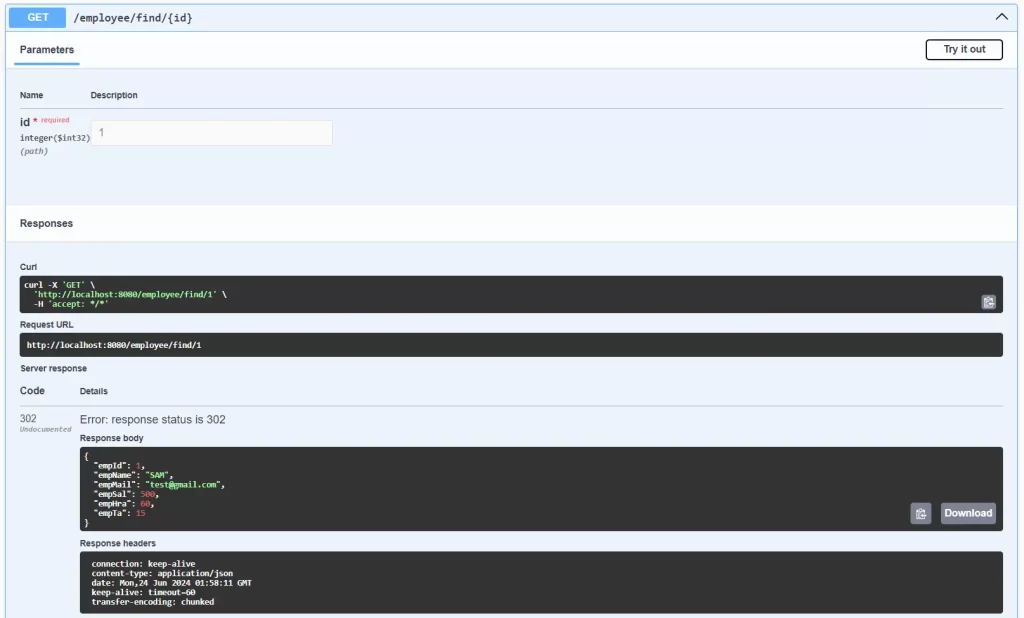
@Tag(name = "Employee", description = "Employee Management APIs")
@RestController
@RequestMapping("/emp")
public class EmployeeController {
@Autowired
EmployeeService employeeService;
/**
* POST - /emp
*
* Save Employee
*
* @param employee
* @return
*/
@Operation(summary = "Save Employee", description = "Create a new employee")
@ApiResponses(value = {
@ApiResponse(responseCode = "201", description = "Employee created",
content = @Content(mediaType = "application/json",
schema = @Schema(implementation = Employee.class))),
@ApiResponse(responseCode = "400", description = "Invalid input")
})
@PostMapping
public ResponseEntity<Employee> save(@RequestBody Employee employee) {
return new ResponseEntity<>(employeeService.save(employee), HttpStatus.CREATED);
}
/**
* PUT - /emp/{id}
*
* Update Employee
*
* @param employee
* @return
*/
@Operation(summary = "Update Employee", description = "Update an existing employee")
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Employee updated",
content = @Content(mediaType = "application/json",
schema = @Schema(implementation = Employee.class))),
@ApiResponse(responseCode = "404", description = "Employee not found")
})
@PutMapping("{id}")
public ResponseEntity<Employee> update(@RequestBody Employee employee, @PathVariable Long id) {
return new ResponseEntity<>(employeeService.update(employee, id), HttpStatus.OK);
}
/**
* GET - /emp
*
* Get All Employees
*
* @return
*/
@Operation(summary = "Get All Employees", description = "Retrieve all employees")
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Employees retrieved",
content = @Content(mediaType = "application/json",
schema = @Schema(implementation = Employee.class)))
})
@GetMapping
public ResponseEntity<List<Employee>> getAllEmployees() {
return ResponseEntity.ok(employeeService.getAll());
}
/**
* GET - /emp/{id}
*
* Get Employee by ID
*
* @param id
* @return
*/
@Operation(summary = "Get Employee by ID",
description = "Retrieve an employee by their ID")
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Employee retrieved",
content = @Content(mediaType = "application/json",
schema = @Schema(implementation = Employee.class))),
@ApiResponse(responseCode = "404", description = "Employee not found")
})
@GetMapping("/{id}")
public ResponseEntity<Employee> getEmployeeById(@PathVariable Long id) {
return ResponseEntity.ok(employeeService.findById(id));
}
/**
* DELETE - /emp/{id}
*
* Delete Employee by ID
*
* @param id
* @return
*/
@Operation(summary = "Delete Employee by ID",
description = "Delete an employee by their ID")
@ApiResponses(value = {
@ApiResponse(responseCode = "202", description = "Employee deleted"),
@ApiResponse(responseCode = "404", description = "Employee not found") })
@DeleteMapping("/{id}")
public ResponseEntity<Void> deleteEmployeeById(@PathVariable Long id) {
employeeService.deleteById(id);
return new ResponseEntity<>(HttpStatus.ACCEPTED);
}
}
GitHub Link:- Spring Rest Swagger Example
Spring Security
Swagger Configuration:-
package com.example.demo.config;
import io.swagger.v3.oas.annotations.OpenAPIDefinition;
import io.swagger.v3.oas.annotations.enums.SecuritySchemeIn;
import io.swagger.v3.oas.annotations.enums.SecuritySchemeType;
import io.swagger.v3.oas.annotations.info.Contact;
import io.swagger.v3.oas.annotations.info.Info;
import io.swagger.v3.oas.annotations.info.License;
import io.swagger.v3.oas.annotations.security.SecurityRequirement;
import io.swagger.v3.oas.annotations.security.SecurityScheme;
@OpenAPIDefinition(
info = @Info(
title = "Know Program",
description = "This API is for User Info",
summary = "API Contains Summary Info",
termsOfService = "https://www.knowprogram.com/terms-and-conditions/",
contact = @Contact(
name="Rocco Jerry",
email="[email protected]",
url="https://www.knowprogram.com"
),
license = @License(name="KnowProgram License"),
version = "API/V1"
),
security = @SecurityRequirement(name="KnowProgramSecurity")
)
@SecurityScheme(
name="KnowProgramSecurity",
in = SecuritySchemeIn.HEADER,
type = SecuritySchemeType.HTTP,
bearerFormat = "Berear ",
description = "This is My Basic Security"
)
public class SwaggerConfig {
}
In Security Config, allow "v3/api-docs/**"
and "/swagger-ui/**"
URLs:-
public static final String[] PUBLIC_URLS = {
"/user/save", "/user/login",
// swagger related URLs
"/v3/api-docs/**", "/swagger-ui/**",
};
@Bean
SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
return http
.csrf(csrf -> csrf.disable())
.authorizeHttpRequests(auth -> {
auth.requestMatchers(PUBLIC_URLS).permitAll()
.anyRequest().authenticated();
})
// other
.build();
}
GitHub Code:- SpringSecurityJWTMySQLSwagger
However, Swagger 2 doesn’t seem to support authentication:- httpAuth HTTP authentication: unsupported scheme ‘token’. It suggests using Swagger solely for documentation purposes, and to send requests to use Postman.
If you enjoyed this post, share it with your friends. Do you want to share more information about the topic discussed above or do you find anything incorrect? Let us know in the comments. Thank you!